Vimeo Player API

The Vimeo Player API allows you to interact with and control an embedded Vimeo Player.
Installation
You can install the Vimeo Player API through either npm:
npm install @vimeo/player
Alternatively, you can reference an up‐to‐date version on our CDN:
<script src="https://player.vimeo.com/api/player.js"></script>
Warning: when used with RequireJS it's required to load the script dynamically via the RequireJS load system. http://www.requirejs.org/docs/api.html#jsfiles
Getting Started
In order to control the Vimeo player, you need a player to control. There are a few ways to get a player:
Pre-existing player
Already have a player on the page? Pass the element to the Vimeo.Player
constructor and you’re ready to go.
<iframe src="https://player.vimeo.com/video/76979871?h=8272103f6e" width="640" height="360" frameborder="0" allowfullscreen allow="autoplay; encrypted-media"></iframe> <script src="https://player.vimeo.com/api/player.js"></script> <script> const iframe = document.querySelector('iframe'); const player = new Vimeo.Player(iframe); player.on('play', function() { console.log('played the video!'); }); player.getVideoTitle().then(function(title) { console.log('title:', title); }); </script>
Create with a video id or url
You can use the library to make the embed for you. All you need is an empty element and the video id or vimeo.com url (and optional embed options).
NOTE: If the video privacy settings are "Unlisted", instead of providing an id
property, you will need to provide the full video URL as a url
property and include the h
parameter.
<div id="made-in-ny"></div> <script src="https://player.vimeo.com/api/player.js"></script> <script> const options = { id: 59777392, width: 640, loop: true }; const player = new Vimeo.Player('made-in-ny', options); player.setVolume(0); player.on('play', function() { console.log('played the video!'); }); </script>
Automatically with HTML attributes
When the library loads, it will scan your page for elements with Vimeo
attributes. Each element must have at least a data-vimeo-id
or
data-vimeo-url
attribute in order for the embed to be created automatically.
You can also add attributes for any of the embed options,
prefixed with data-vimeo
(data-vimeo-portrait="false"
, for example).
NOTE: If the video privacy settings are "Unlisted", instead of providing a data-vimeo-id
attribute, you will need to provide the full video URL in a data-vimeo-url
attribute and include the h
parameter.
<div data-vimeo-id="19231868" data-vimeo-width="640" id="handstick"></div> <div data-vimeo-url="https://player.vimeo.com/video/76979871?h=8272103f6e" id="playertwo"></div> <script src="https://player.vimeo.com/api/player.js"></script> <script> // If you want to control the embeds, you’ll need to create a Player object. // You can pass either the `<div>` or the `<iframe>` created inside the div. const handstickPlayer = new Vimeo.Player('handstick'); handstickPlayer.on('play', function() { console.log('played the handstick video!'); }); const playerTwoPlayer = new Vimeo.Player('playertwo'); playerTwoPlayer.on('play', function() { console.log('played the player 2.0 video!'); }); </script>
Browser Support
The Player API library is supported in IE 11+, Chrome, Firefox, Safari, and Opera.
Migrate from Froogaloop
Using our old Froogaloop library? See the migration doc for details on how to update your code to use this library.
Using with a module bundler
If you’re using a module bundler like webpack or
rollup, the exported object will be the Player
constructor (unlike the browser where it is attached to window.Vimeo
):
import Player from '@vimeo/player'; const player = new Player('handstick', { id: 19231868, width: 640 }); player.on('play', function() { console.log('played the video!'); });
Similarly, if you’re using RequireJS in the browser, it will also import the Player constructor directly:
<iframe src="https://player.vimeo.com/video/76979871?h=8272103f6e" width="640" height="360" frameborder="0" allowfullscreen allow="autoplay; encrypted-media"></iframe> <script> require(['https://player.vimeo.com/api/player.js'], function (Player) { const iframe = document.querySelector('iframe'); const player = new Player(iframe); player.on('play', function() { console.log('played the video!'); }); }); </script>
Table of Contents
- Create a Player
- Embed Options
- Methods
- on
- off
- loadVideo
- ready
- enableTextTrack
- disableTextTrack
- pause
- play
- unload
- destroy
- requestFullscreen
- exitFullscreen
- getFullscreen
- requestPictureInPicture
- exitPictureInPicture
- getPictureInPicture
- remotePlaybackPrompt
- getRemotePlaybackAvailability
- getRemotePlaybackState
- getAutopause
- setAutopause
- getBuffered
- getChapters
- getCurrentChapter
- getColor
- getColors
- setColor
- setColors
- addCuePoint
- removeCuePoint
- getCuePoints
- getCurrentTime
- setCurrentTime
- getDuration
- getEnded
- getLoop
- setLoop
- getMuted
- setMuted
- getPaused
- getPlaybackRate
- setPlaybackRate
- getPlayed
- getSeekable
- getSeeking
- getTextTracks
- getVideoEmbedCode
- getVideoId
- getVideoTitle
- getVideoWidth
- getVideoHeight
- getVideoUrl
- getVolume
- setVolume
- setTimingSrc
- getQualities
- getQuality
- setQuality
- getCameraProps
- setCameraProps
- Events
- play
- playing
- pause
- ended
- timeupdate
- progress
- seeking
- seeked
- texttrackchange
- chapterchange
- cuechange
- cuepoint
- volumechange
- playbackratechange
- bufferstart
- bufferend
- error
- loaded
- durationchange
- fullscreenchange
- qualitychange
- camerachange
- resize
- enterpictureinpicture
- leavepictureinpicture
- remoteplaybackavailabilitychange
- remoteplaybackconnecting
- remoteplaybackconnect
- remoteplaybackdisconnect
- interactivehotspotclicked
- interactiveoverlaypanelclicked
Create a Player
The Vimeo.Player
object wraps an iframe so you can interact with and control a
Vimeo Player embed.
Existing embed
If you already have a Vimeo <iframe>
on your page, pass that element into the
constructor to get a Player
object. You can also use jQuery to select the
element, or pass a string that matches the id
of the <iframe>
.
// Select with the DOM API const iframe = document.querySelector('iframe'); const iframePlayer = new Vimeo.Player(iframe); // Select with jQuery // If multiple elements are selected, it will use the first element. const jqueryPlayer = new Vimeo.Player($('iframe')); // Select with the `<iframe>`’s id // Assumes that there is an <iframe id="player1"> on the page. const idPlayer = new Vimeo.Player('player1');
Create an embed
Pass any element and an options object to the Vimeo.Player
constructor to make
an embed inside that element. The options object should consist of either an
id
or url
and any other embed options for the embed.
NOTE: If the video privacy settings are "Unlisted", instead of providing an id
property, you will need to provide the full video URL as a url
property and include the h
parameter.
<div id="made-in-ny"></div> <script src="https://player.vimeo.com/api/player.js"></script> <script> const options = { id: 59777392, width: 640, loop: true }; // Will create inside the made-in-ny div: // <iframe src="https://player.vimeo.com/video/59777392?h=ab882a04fd&loop=1" width="640" height="360" frameborder="0" allowfullscreen allow="autoplay; encrypted-media"></iframe> const madeInNy = new Vimeo.Player('made-in-ny', options); </script>
Embed options will also be read from the data-vimeo-*
attributes. Attributes
on the element will override any defined in the options object passed to the
constructor (similar to how the style
attribute overrides styles defined in
CSS).
Elements with a data-vimeo-id
or data-vimeo-url
attribute will have embeds
created automatically when the player API library is loaded. You can use the
data-vimeo-defer
attribute to prevent that from happening and create the embed
at a later time. This is useful for situations where the player embed wouldn’t
be visible right away, but only after some action was taken by the user (a
lightbox opened from clicking on a thumbnail, for example).
<div data-vimeo-id="59777392" data-vimeo-defer id="made-in-ny"></div> <div data-vimeo-id="19231868" data-vimeo-defer data-vimeo-width="500" id="handstick"></div> <script src="https://player.vimeo.com/api/player.js"></script> <script> const options = { width: 640, loop: true }; // Will create inside the made-in-ny div: // <iframe src="https://player.vimeo.com/video/59777392?h=ab882a04fd&loop=1" width="640" height="360" frameborder="0" allowfullscreen allow="autoplay; encrypted-media"></iframe> const madeInNy = new Vimeo.Player('made-in-ny', options); // Will create inside the handstick div: // <iframe src="https://player.vimeo.com/video/19231868?h=1034d5269b&loop=1" width="500" height="281" frameborder="0" allowfullscreen allow="autoplay; encrypted-media"></iframe> const handstick = new Vimeo.Player(document.getElementById('handstick'), options); </script>
Embed Options
These options are available to be appended to the query string of the player URL, used as data-vimeo-
attributes on elements, or included as
an object passed to the Vimeo.Player
constructor. The complete list of embed options can be found in our official SDK documentation.
Methods
You can call methods on the player by calling the function on the Player object:
player.play();
All methods, except for on()
and off()
return a
Promise. The Promise may
or may not resolve with a value, depending on the specific method.
player.disableTextTrack().then(function() { // the track was disabled }).catch(function(error) { // an error occurred });
Promises for getters are resolved with the value of the property:
player.getLoop().then(function(loop) { // whether or not the player is set to loop });
Promises for setters are resolved with the value set, or rejected with an error if the set fails. For example:
player.setColor('#00adef').then(function(color) { // the color that was set }).catch(function(error) { // an error occurred setting the color });
on(event: string, callback: function): void
Add an event listener for the specified event. Will call the callback with a
single parameter, data
, that contains the data for that event. See
events below for
编辑推荐精选
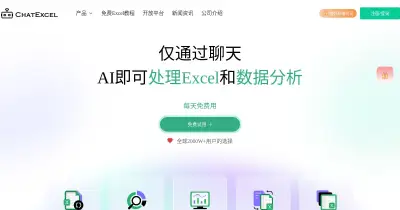
酷表ChatExcel
大模型驱动的Excel数据处理工具
基于大模型交互的表格处理系统,允许用户通过对话方式完成数据整理和可视化分析。系统采用机器学习算法解析用户指令,自动执行排序、公式计算和数据透视等操作,支持多种文件格式导入导出。数据处理响应速度保持在0.8秒以内,支持超过100万行数据的即时分析。
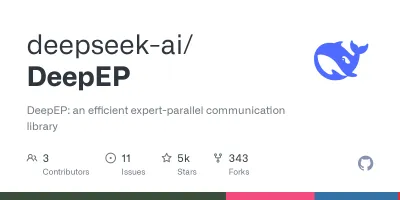

DeepEP
DeepSeek开源的专家并行通信优化框架
DeepEP是一个专为大规模分布式计算设计的通信库,重点解决专家并行模式中的通信瓶颈问题。其核心架构采用分层拓扑感知技术,能够自动识别节点间物理连接关系,优化数据传输路径。通过实现动态路由选择与负载均衡机制,系统在千卡级计算集群中维持稳定的低延迟特性,同时兼容主流深度学习框架的通信接口。
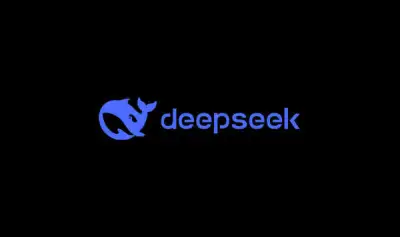

DeepSeek
全球领先开源大模型,高效智能助手
DeepSeek是一家幻方量化创办的专注于通用人工智能的中国科技公司,主攻大模型研发与应用。DeepSeek-R1是开源的推理模型,擅长处理复杂任务且可免费商用。
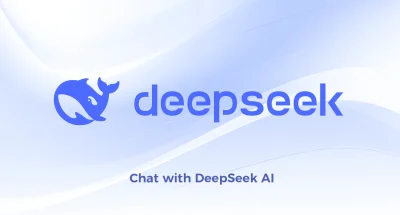

问小白
DeepSeek R1 满血模型上线
问小白是一个基于 DeepSeek R1 模型的智能对话平台,专为用户提供高效、贴心的对话体验。实时在线,支持深度思考和联网搜索。免费不限次数,帮用户写作、创作、分析和规划,各种任务随时完成!
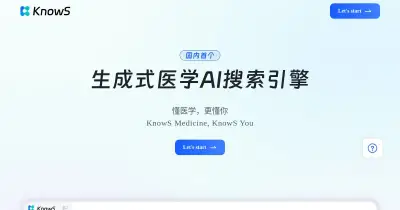

KnowS
AI医学搜索引擎 整合4000万+实时更新的全球医学文献
医学领域专用搜索引擎整合4000万+实时更新的全球医学文献,通过自主研发AI模型实现精准知识检索。系统每日更新指南、中英文文献及会议资料,搜索准确率较传统工具提升80%,同时将大模型幻觉率控制在8%以下。支持临床建议生成、文献深度解析、学术报告制作等全流程科研辅助,典型用户反馈显示每周可节省医疗工作者70%时间。
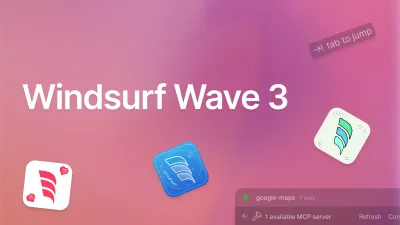

Windsurf Wave 3
Windsurf Editor推出第三次重大更新Wave 3
新增模型上下文协议支持与智能编辑功能。本次更新包含五项核心改进:支持接 入MCP协议扩展工具生态,Tab键智能跳转提升编码效率,Turbo模式实现自动化终端操作,图片拖拽功能优化多模态交互,以及面向付费用户的个性化图标定制。系统同步集成DeepSeek、Gemini等新模型,并通过信用点数机制实现差异化的资源调配。
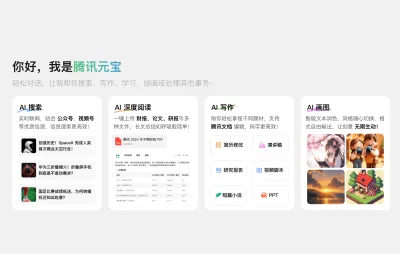

腾讯元宝
腾讯自研的混元大模型AI助手
腾讯元宝是腾讯基于自研的混元大模型推出的一款多功能AI应用,旨在通过人工智能技术提升用户在写作、绘画、翻译、编程、搜索、阅读总结等多个领域的工作与生活效率。
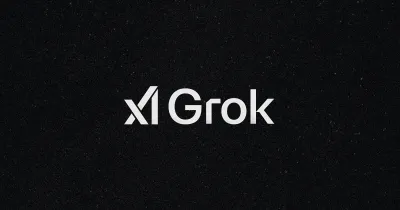

Grok3
埃隆·马斯克旗下的人工智能公司 xAI 推出的第三代大规模语言模型
Grok3 是由埃隆·马斯克旗下的人工智能公司 xAI 推出的第三代大规模语言模型,常被马斯克称为“地球上最聪明的 AI”。它不仅是在前代产品 Grok 1 和 Grok 2 基础上的一次飞跃,还在多个关键技术上实现了创新突破。
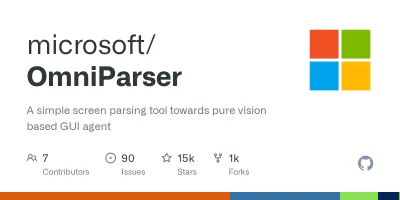

OmniParser
帮助AI理解电脑屏幕 纯视觉GUI元素的自动化解析方案
开源工具通过计算机视觉技术实现图形界面元素的智能识别与结构化处理,支持自动化测试脚本生成和辅助功能开发。项目采用模块化设计,提供API接口与多种输出格式,适用于跨平台应用场景。核心算法优化了元素定位精度,在动态界面和复杂布局场景下保持稳定解析能力。
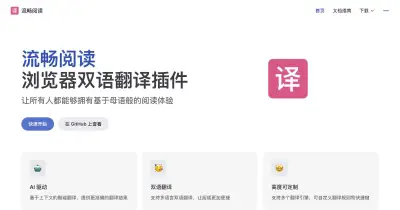

流畅阅读
AI网页翻译插件 双语阅读工具,还原母语级体验
流畅阅读是一款浏览器翻译插件,通过上下文智能分析提升翻译准确性,支持中英双语对照显示。集成多翻译引擎接口,允许用户自定义翻译规则和快捷键配置,操作数据全部存储在本地设备保障隐私安全。兼容Chrome、Edge、Firefox等主流浏览器,基于GPL-3.0开源协议开发,提供持续的功能迭代和社区支持。
推荐工具精选
AI云服务特惠
懂AI专属折扣关注微信公众号
最新AI工具、AI资讯
独家AI资源、AI项目落地
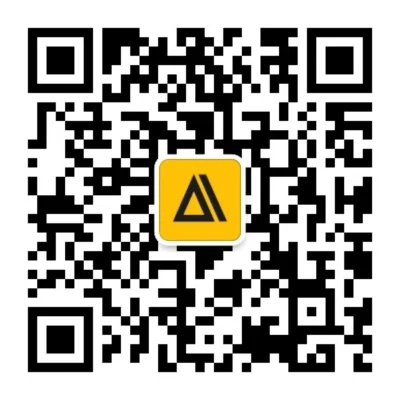
微信扫一扫关注公众号