React+TypeScript Cheatsheets
Cheatsheets for experienced React developers getting started with TypeScript
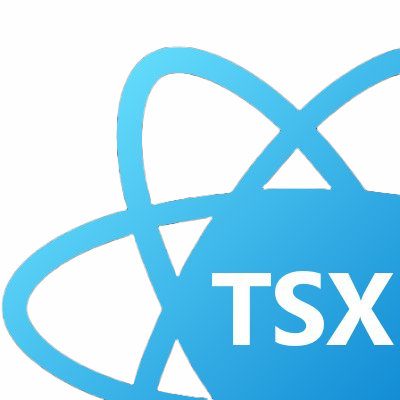
Web docs | Español | Português | Contribute! | Ask!
:wave: This repo is maintained by @swyx, @eps1lon and @filiptammergard. We're so happy you want to try out TypeScript with React! If you see anything wrong or missing, please file an issue! :+1:
All React + TypeScript Cheatsheets
- The Basic Cheatsheet is focused on helping React devs just start using TS in React apps
- Focus on opinionated best practices, copy+pastable examples.
- Explains some basic TS types usage and setup along the way.
- Answers the most Frequently Asked Questions.
- Does not cover generic type logic in detail. Instead we prefer to teach simple troubleshooting techniques for newbies.
- The goal is to get effective with TS without learning too much TS.
- The Advanced Cheatsheet helps show and explain advanced usage of generic types for people writing reusable type utilities/functions/render prop/higher order components and TS+React libraries.
- It also has miscellaneous tips and tricks for pro users.
- Advice for contributing to DefinitelyTyped.
- The goal is to take full advantage of TypeScript.
- The Migrating Cheatsheet helps collate advice for incrementally migrating large codebases from JS or Flow, from people who have done it.
- We do not try to convince people to switch, only to help people who have already decided.
- ⚠️This is a new cheatsheet, all assistance is welcome.
- The HOC Cheatsheet) specifically teaches people to write HOCs with examples.
- Familiarity with Generics is necessary.
- ⚠️This is the newest cheatsheet, all assistance is welcome.
Basic Cheatsheet
Basic Cheatsheet Table of Contents
Expand Table of Contents
-
- Function Components
- Hooks
- useState
- useReducer
- useEffect / useLayoutEffect
- useRef
- useImperativeHandle
- Custom Hooks
- More Hooks + TypeScript reading:
- Example React Hooks + TypeScript Libraries:
- Class Components
- Typing getDerivedStateFromProps
- You May Not Need
defaultProps
- Typing
defaultProps
- Consuming Props of a Component with defaultProps
- Misc Discussions and Knowledge
- Typing Component Props
- Basic Prop Types Examples
- Useful React Prop Type Examples
- Types or Interfaces?
-
Troubleshooting Handbook: Globals, Images and other non-TS files
Section 1: Setup
Prerequisites
You can use this cheatsheet for reference at any skill level, but basic understanding of React and TypeScript is assumed. Here is a list of prerequisites:
- Basic understanding of React.
- Familiarity with TypeScript Basics and Everyday Types.
In the cheatsheet we assume you are using the latest versions of React and TypeScript.
React and TypeScript starter kits
React has documentation for how to start a new React project with some of the most popular frameworks. Here's how to start them with TypeScript:
- Next.js:
npx create-next-app@latest --ts
- Remix:
npx create-remix@latest
- Gatsby:
npm init gatsby --ts
- Expo:
npx create-expo-app -t with-typescript
Try React and TypeScript online
There are some tools that let you run React and TypeScript online, which can be helpful for debugging or making sharable reproductions.
Section 2: Getting Started
Function Components
These can be written as normal functions that take a props
argument and return a JSX element.
// Declaring type of props - see "Typing Component Props" for more examples
type AppProps = {
message: string;
}; /* use `interface` if exporting so that consumers can extend */
// Easiest way to declare a Function Component; return type is inferred.
const App = ({ message }: AppProps) => <div>{message}</div>;
// You can choose to annotate the return type so an error is raised if you accidentally return some other type
const App = ({ message }: AppProps): React.JSX.Element => <div>{message}</div>;
// You can also inline the type declaration; eliminates naming the prop types, but looks repetitive
const App = ({ message }: { message: string }) => <div>{message}</div>;
// Alternatively, you can use `React.FunctionComponent` (or `React.FC`), if you prefer.
// With latest React types and TypeScript 5.1. it's mostly a stylistic choice, otherwise discouraged.
const App: React.FunctionComponent<{ message: string }> = ({ message }) => (
<div>{message}</div>
);
// or
const App: React.FC<AppProps> = ({ message }) => <div>{message}</div>;
Tip: You might use Paul Shen's VS Code Extension to automate the type destructure declaration (incl a keyboard shortcut).
Why is React.FC
not needed? What about React.FunctionComponent
/React.VoidFunctionComponent
?
You may see this in many React+TypeScript codebases:
const App: React.FunctionComponent<{ message: string }> = ({ message }) => (
<div>{message}</div>
);
However, the general consensus today is that React.FunctionComponent
(or the shorthand React.FC
) is not needed. If you're still using React 17 or TypeScript lower than 5.1, it is even discouraged. This is a nuanced opinion of course, but if you agree and want to remove React.FC
from your codebase, you can use this jscodeshift codemod.
Some differences from the "normal function" version:
-
React.FunctionComponent
is explicit about the return type, while the normal function version is implicit (or else needs additional annotation). -
It provides typechecking and autocomplete for static properties like
displayName
,propTypes
, anddefaultProps
.- Note that there are some known issues using
defaultProps
withReact.FunctionComponent
. See this issue for details. We maintain a separatedefaultProps
section you can also look up.
- Note that there are some known issues using
-
Before the React 18 type updates,
React.FunctionComponent
provided an implicit definition ofchildren
(see below), which was heavily debated and is one of the reasonsReact.FC
was removed from the Create React App TypeScript template.
// before React 18 types
const Title: React.FunctionComponent<{ title: string }> = ({
children,
title,
}) => <div title={title}>{children}</div>;
(Deprecated)Using React.VoidFunctionComponent
or React.VFC
instead
In @types/react 16.9.48, the React.VoidFunctionComponent
or React.VFC
type was added for typing children
explicitly.
However, please be aware that React.VFC
and React.VoidFunctionComponent
were deprecated in React 18 (https://github.com/DefinitelyTyped/DefinitelyTyped/pull/59882), so this interim solution is no longer necessary or recommended in React 18+.
Please use regular function components or React.FC
instead.
type Props = { foo: string };
// OK now, in future, error
const FunctionComponent: React.FunctionComponent<Props> = ({
foo,
children,
}: Props) => {
return (
<div>
{foo} {children}
</div>
); // OK
};
// Error now, in future, deprecated
const VoidFunctionComponent: React.VoidFunctionComponent<Props> = ({
foo,
children,
}) => {